JS itself provides us with many objects such as Array, Date, and Math, but in actual development, we still use custom objects the most. Custom objects are a subject worth studying. To put it simply, it is related to code volume, encapsulation, and whether the code is elegant; Going deeper, it involves memory overhead, design patterns, and even the core of JavaScript language. Let’s take a step-by-step look at how to better create an object.
Basic mode
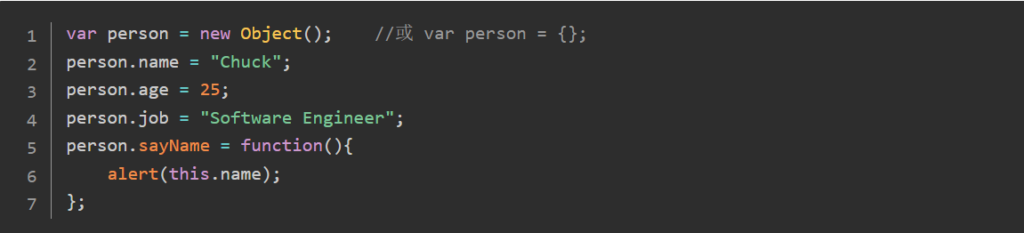
This is a common way for us to create an object, which is very natural and easy to write, but don’t you think it’s a bit troublesome to write 5 people in a row? So we came up with the idea of using object literal syntax for improvement.
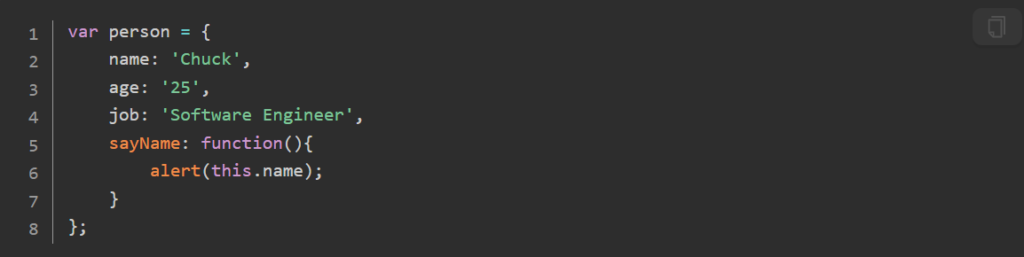
Is this much better? Yes, literal grammar is really a good thing! Indeed, using object literal form to create objects is a very common and popular way, as it is convenient when only a few objects need to be defined separately. But the problem is, when I want to create many objects with the same structure as the person mentioned above, this solution is not very good. Do I have to type the name age job sayName every time I create an object? This is obviously unrealistic and will result in a large amount of duplicate code.
Factory mode
The above question is that we don’t want to completely manually assemble every object, but rather want something like a mold. We just need to throw different materials in, and what comes out is the object we want, which has the same structure but different content. This mold is like a factory, and we use functions to build this “factory”:
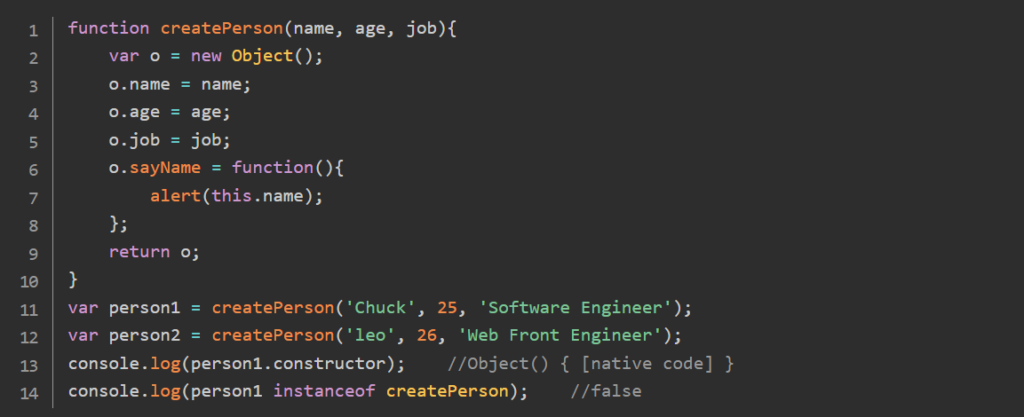
This createPerson() function is a “factory” that allows us to easily create as many objects as we want, as long as we “throw materials” into it.
Constructor Pattern
What is a constructor? We know that in JavaScript, the Object () in var o=new Object () is a native constructor that can construct objects of type Object. Similarly, Array() and Date() are native constructors provided by JS, which can construct array objects and date objects respectively. In addition to these native constructors, we can also customize constructors for us to use:
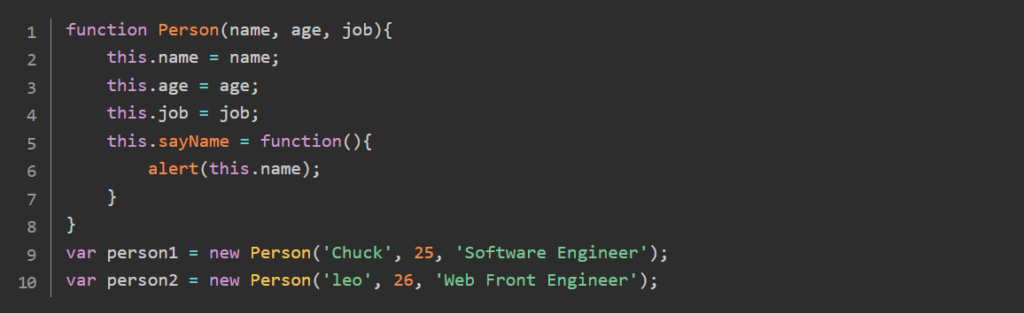
The only difference between constructors and other functions is the way they are called. However, constructors are also functions, and there is no special syntax for defining constructors. Any function that can be called through the new operator can serve as a constructor; And any function, if not called through the new operator, will not be much different from a regular function.
Conclusion
The above modes of creating objects are progressive from inferior to superior, hoping to provide some help to beginners.